Disclaimer: from now on I will occasionally post in English too.
A first release of an Wheel of Fortune made with JavaFX. There’s still has a lot of bugs but is already usable. Let’s say that version is 0.8. 🙂
import javafx.ui.*; import javafx.ui.canvas.*; import javafx.ui.filter.*; import java.util.Random; import java.lang.System; class Wheel extends CompositeNode{ attribute n: Integer; attribute radius: Integer; attribute cx: Integer; attribute cy: Integer; attribute colors: Color*; private attribute angle: Number; private attribute rotAngle: Number; private attribute extraTurns: Integer; operation run(); } attribute Wheel.n = 32; attribute Wheel.radius = 100; attribute Wheel.cx = radius; attribute Wheel.cy = radius; attribute Wheel.rotAngle = 0; attribute Wheel.angle = bind 360/n; attribute Wheel.extraTurns = 2; attribute Wheel.colors = [ red:Color, lime:Color, blue:Color, yellow:Color, orange:Color, cyan:Color ]; function Wheel.composeNode(){ var selector = Rect { x: radius*2 - 10 y: radius width: 30 height: 10 fill:black }; var disc = foreach(j in [1..n]) Arc{ transform: translate(-radius, -radius) width: radius * 2 height: radius * 2 startAngle: j*angle length: angle fill:colors[j%(sizeof colors)] closure: PIE }; var border = Circle{ radius:radius stroke:gray strokeWidth: 2 }; var glass = Circle{ radius:radius - 25 fill: white opacity: 0.3 }; var star = Star { rin: radius/4 rout: radius/2 points: 5 startAngle: 18 fill: yellow }; var numbers = foreach(i in [1..n]) Text { transform: [rotate(i*angle, 0, 0), translate(radius - 20,0)] content: i.toString() }; var base = Group{ transform: bind [rotate(rotAngle,cx,cy),translate(cx,cy),] content: [disc, border, glass, numbers, star] }; return Group { content: [selector, base] }; } operation Wheel.run() { var fortune = new Random(System.currentTimeMillis()); var chosen = fortune.nextInt(n); System.out.println(chosen); rotAngle = [1.. extraTurns*360+ chosen*angle] dur 200 * n; } var wheel = Wheel{}; Frame{ visible: true content: Canvas { content: [ wheel, View { content:Button { text: "Go" action: operation() { wheel.run(); } } } ] } }
The JavaFX Wheel of Fortune in use:
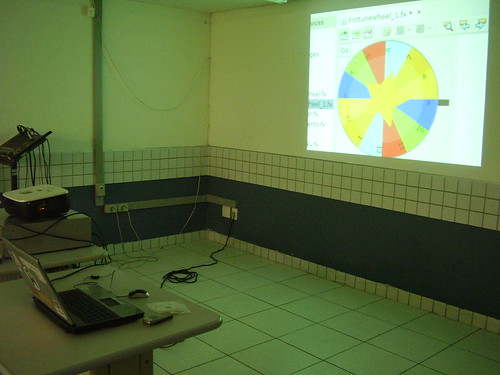
- ps: this code was actualized. Now it’s using colors constants and time as random seed.
- ps2: this code and video has figured out in the front page of the Project OpenJFX! Thank you guys.
- ps3: In 03/15/2008 I modified this code. Now you can put an arbitrary number for Wheel.n and the wheel should render good.
[…] used the JavaFX Whell of Fortune for give […]
[…] Do you remember our Wheel of Fortune in JavaFX? […]
After some changes in the code, it works very well in NB6.5 and it is just cool! thanks.
Hello,
I am at present in 1st year in computing. To make a success of the year I have to program a game as the wheel of fortune. I have already made everything, but I still have the wheel to be made and I do not manage to find a method to make it. Your code corresponds exactly to the fact that I look but as I do not know the language JavaFx then I wanted to know if you also have it in Javascripte, please ?
Thanks.
Hi Bao Long Ngo Vuong, unfortunately this code posted in this post is deprecated.
You can use the idea showed here but need to rewrite it on compliance with a newer JavaFX version. In this moment I’m writing the latest version is the 1.1
No, I don’t have a JavaScript version, sorry.