Continuing my little JavaFX framework for game development, right now focused on use those tiles I’m drawing and posting here in my blog. This framework will be a group of classes for simplify and hide some complexities of common game development. Right now I wrote just a few of them.
Use
We create a tileset from the files.png file that way
var tileset = Tileset {
cols: 15 rows: 10 height: 32 width: 32
image: Image {
url: "{__DIR__}tiles.png"
}
}
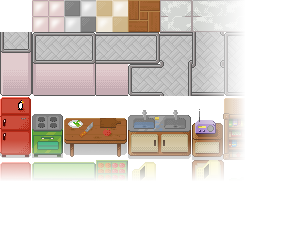
Tileset are orthogonal, distributed into a grid of cols columns and rows rows. Each tile have dimensions height x width.
A Tileset is used into a Tilemap
var bg = Tilemap {
set:tileset cols:5 rows:5
map: [8,8,8,8,8,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3]
}
That shows
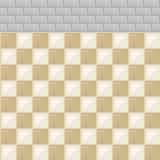
Each number in the map represents a tile in the tilemap. Number 0 means the first tile at the upper left corner, numbers keep growing from left to right columns, from top to bottom rows.
Another example
var things = Tilemap {
set:tileset cols:5 rows:5
map: [80,55,56,145,145,96,71,72,61,62,0,0,0,77,78,122,0,0,93,94,138,0,0,0,0]
}
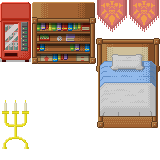
A tilemap can also contains more than one layer
var room = Tilemap {
set:tileset cols:5 rows:5 layers:2
map: [
[8,8,8,8,8,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3,3],
[80,55,56,145,145,96,71,72,61,62,0,0,0,77,78,122,0,0,93,94,138,0,0,0,0]
]
}
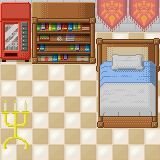
Implementation
The Tileset class basically stores a Image and a collection of Rectangle2D objects, for be used as viewports in ImageView classes.
import javafx.scene.image.ImageView;
import javafx.scene.image.Image;
import javafx.geometry.Rectangle2D;
public class Tileset {
public-init var image: Image;
public-init var width: Integer = 32;
public-init var height: Integer = 32;
public-init var rows: Integer = 10;
public-init var cols: Integer = 15;
protected var tile: Rectangle2D[];
init {
tile = for (row in [0..rows]) {
for (col in [0..cols]) {
Rectangle2D{
minX: col * width, minY: row * height
height: width, width: height
}
}
}
}
}
The Tilemap is a CustomNode with a Group of ImageViews in a grid. The grid is mounted by iterating over the map as many layers was defined.
public class Tilemap extends CustomNode {
public-init var rows: Integer = 10;
public-init var cols: Integer = 10;
public-init var set: Tileset;
public-init var layers: Integer = 1;
public-init var map: Integer[];
public override function create(): Node {
var tilesperlayer = rows * cols;
return Group {
content:
for (layer in [0..layers]) {
for (row in [0..rows-1]) {
for (col in [0..cols-1]) {
ImageView {
image: set.image x: col * set.width y: row * set.height
viewport: set.tile[map[tilesperlayer*layer + row*rows+col]]
}
}
}
}
};
}
}
Next steps
- Integrate to a map editor
- Support some XML map format
- Sprite classes for animation
- Integrate those collision detection classes I posted before
Download